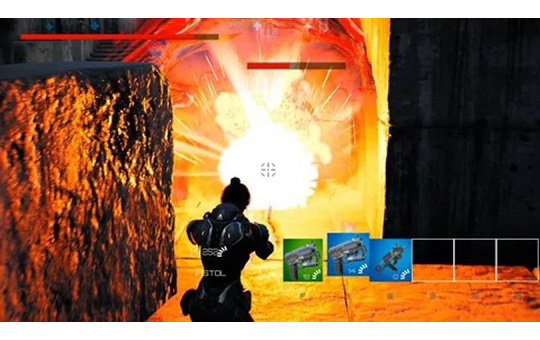
Unreal Engine C++ The Ultimate Shooter Course
Master Unreal Engine by Creating a Complete Shooter Game!
The course is compatible with any version of Unreal Engine, including Unreal Engine 5!
This is the most comprehensive Unreal Engine tutorial series on the internet. You will create a shooter game complete with AAA quality gameplay mechanics. Learn the theory behind the gameplay mechanics of shooter games, then implement them in this beautiful game project. Topics covered are:
-
Character creation and movement
-
Input for PC and console controllers
-
Extensive use of Animation Blueprints (and Anim Instances, their C++ parents)
-
1D and 2D Blendspaces
-
Strafing
-
Turn-in-place
-
Aim Offsets
-
Inverse Kinematics
-
Animation Curves
-
Character lean when running
-
Crouching (with dynamic capsule resizing)
-
Turn hips while running
-
Weapon fire with recoil animations
-
Reloading
-
Weapon blast and impact particles
-
Weapon beam particles (smoke trails)
-
Bullet shell eject particles
-
Sound effects
-
Blending animations per bone, by bool, and by enum – play one animation with one part of the body (running or crouching) while simultaneously playing another animation with another part of the body (reload, aim, or weapon fire)
-
Attach and equip different weapons (pistols, submachine guns, assault rifles)
-
Automatic and semi-automatic gunfire
-
Move different gun parts (the clip/magazine, and pistol slide) during animations
-
Camera zoom while aiming
-
Dynamic crosshairs that spread in reaction to:
-
Character speed
-
Weapon fire
-
Aiming
-
Jumping
-
-
Different crosshairs per weapon
-
Widget components, showing:
-
Item names
-
Item types
-
Ammo counts
-
Item rarity
-
-
HUD animations
-
Full item inventory system
-
Data tables, in Blueprints and C++
-
Curves to control:
-
Item movement during pickup
-
Color and brightness pulse for material effects
-
-
Material creation, including:
-
Post-process materials
-
Dynamic material instances
-
Setting material properties from C++
-
Driving material properties with curves
-
Material functions
-
Blending materials together
-
Outline effects
-
Glow/pulse effects
-
-
Retargeting animations
-
Retargeting whole Animation Blueprints
-
Numerous gameplay algorithms
-
Use of data structures, including:
-
structs
-
enums
-
arrays
-
maps
-
and more
-
-
Animation montages
-
Anim Notifies for sounds, weapon trails, and custom notifies
-
Sync markers for footsteps and sync groups
-
The course comes with a huge amount of assets, including:
-
Sounds
-
Textures
-
Particle effects
-
Meshes
-
-
Delegates
-
Interfaces
-
Dynamic footsteps that spawn different sounds and particle systems depending on the surface type
-
Physical materials and surface types
-
Niagra particle systems
-
Line traces
-
Enemy AI
-
Behavior Trees and Blackboard Components
-
Multiple enemy types with varying levels of Health, Damage, Size and Movement Speed
-
Headshot damage, with:
-
Higher damage for headshots
-
Number widgets that pop up and animate with bullet hits
-
Different colored numbers for headshots
-
-
Character and enemy health bars
-
Enemy patrol, agro, chase and attack player
-
Death mechanics
-
Stun mechanics
-
Melee attacks with melee weapon trails
-
Explosives that cause damage and death
-
Level prototyping
-
Creating full levels based on our prototypes using professional assets
-
Light baking
-
Post-process effects
What you’ll learn
- Learn all skills that translate to Unreal Engine 5!
- Learn Unreal Engine game development by creating a complete shooter game.
- Get in-depth experience with Unreal Engine Animation, Materials, Special Effects, and gameplay!
- Learn Unreal Engine’s code base.
Are there any course requirements or prerequisites?
- Understanding of the basics of C++ (take my C++ course!)
Who this course is for:
- Unreal Engine beginners who understand the basics of C++.
- Unreal Engine intermediate developers who wish to advance in skill level.
- Expert Unreal Engine developers to wish to expand their skillset.
- Those who wish to make shooter games.
- Anyone who wants to have fun and make games!
Curriculum
- 17 Sections
- 345 Lessons
- 1 Quiz
- 0m Duration
Section 1: Introduction
- Introduction(5:59)
- Install an IDE(5:53)
- Install Unreal Engine(2:18)
- C++ Refresher(11:47)
- Quiz on C++ Concepts
- Reflection and Garbage Collection(3:24)
- How to Get Help(10:22)
Section 2: Project Setup
- Project Setup(8:37)
- Character Class(5:46)
- UE_LOG Format String - Int
- UE_LOG Format Specifiers(7:43)
- UE_LOG with FString(4:07)
- Camera Spring Arm(13:31)
- Follow Camera(6:48)
- Controllers and Input(4:23)
- Move Forward and Right(16:00)
- Delta Time(6:33)
- Turn at Rate(15:10)
- Mouse Turning and Jumping(7:32)
- Adding a Mesh(4:25)
- Smoothing Character Movement(5:48)
Section 3: Animations
- The Anim Instance(16:58)
- The Animation Blueprint
- Run Animations(10:58)
- Trimming Animations(10:01)
- Rotate Character to Movement(7:21)
- Fire Weapon Function(4:04)
- Shooting Sound Effects(10:22)
- Shooting Particles(13:25)
- Shooting Animation(11:44)
- Blending Shooting Animations(6:21)
- Line Tracing for Bullet Hits(15:40)
- Impact Particles(5:01)
- Beam Particles
- Socket Offset(2:59)
- HUD Class and Crosshairs(11:39)
- Directing Rifle Shots(14:49)
- Trace from Gun Barrel(9:23)
- Refactor Beam End Code(11:19)
- Movement Offset Yaw(16:34)
- Strafing Blendspace(8:33)
- Jog Start Blendspace(10:39)
- Jog Stop Blendspace(11:09)
Section 4: Aiming and Crosshairs
- Zooming Field of View(11:27)
- Aiming Zoom Interpolation(13:28)
- Aiming Pose(7:48)
- Aiming State Machine(8:36)
- Aim Look Sensitivity(20:42)
- Crosshair Spread Velocity(11:20)
- Spreading the Crosshairs(17:43)
- Crosshair In Air Factor(9:09)
- Crosshair Aim Factor(6:34)
- Bullet Fire Aim Factor(15:18)
- New Level Assets(10:01)
- Automatic Fire
- Jumping Animations(12:38)
Section 5: The Weapon
- The Item Class(8:09)
- The Weapon Class(15:18)
- UMG Intro Lesson(6:00)
- PickupWidget Blueprint(19:37)
- Finishing the Pickup Widget(19:15)
- Add Widget to Weapon(4:40)
- Trace for Widget(8:00)
- Refactor Trace Under Crosshairs(12:55)
- Widget Trace When Close(25:31)
- Hide Widget(10:24)
- Bind Item Name(9:04)
- Bind Item Count(7:19)
- Bind Star Opacity(26:30)
- Spawn Default Weapon(15:54)
- Equip Function(9:59)
- Item State Lesson(7:27)
- Item State(5:40)
- Set Item Properties(12:37)
- Detach Weapon(7:35)
- Item Falling State(10:54)
- Throw Weapon(15:50)
- Swap Weapon(12:13)
Section 6: Item Interpolation
- Item Interping Slide(10:05)
- Camera Interp Location(8:43)
- Get Pickup Item(3:09)
- Item Z Curve(8:16)
- Item Interp Variables(13:01)
- Interping State Properties(3:02)
- Following the Z Curve
- Interp Item X and Y(9:03)
- Interp Rotation(10:48)
- Interp Scale(13:32)
Section 7: Reloading
- Retargeting Animations(12:03)
- Edit Animations in Unreal(11:46)
- Ammo(11:07)
- 5. Ammo Count Widget(13:13)
- Draw Ammo Count to Screen(15:20)
- Weapon Ammo in C++(8:20)
- Bind Weapon Ammo in Widget(4:35)
- Fixing Barrel Socket Location(4:59)
- Improving Weapon Fire Code Lecture(13:04)
- Improving Weapon Fire Code(15:53)
- Reload Montage(5:42)
- Reload Lecture(15:50)
- The Weapon Type(3:43)
- Reload Continued(17:24)
- Update AmmoMap(15:33)
- Bind Carried Ammo(7:22)
- Bind Weapon Name(3:51)
- Move Clip Lecture(7:46)
- Grab and Release Clip(19:20)
- Weapon Anim BP(11:59)
- Moving the Clip(15:00)
- Clip Sounds(10:07)
- Pickup Sounds(10:12)
Section 8: Advanced Movement
- Rotate Root Bone(22:24)
- Turn in Place Animations(16:49)
- Animation Curves(9:31)
- Turn in Place using Curve Values(19:14)
- Hip Aim Offset(25:17)
- Aiming Aim Offset(21:14)
- Lean(16:09)
- Lean Blendspace(14:35)
- Crouching Setup(6:52)
- Crouching Animations(7:27)
- Crouching AnimBP(6:17)
- Crouching Turn Animations(10:04)
- Retargeting Anims with Different Skeletons(20:59)
- Crouch Turn in Place Anim BP(19:40)
- Crouch Recoil Weight(12:51)
- Crouch Walking Blendspace(7:13)
- Crouch Walking(5:52)
- Crouch Movement Speed and Jump(7:03)
- Interp Capsule Half Height(22:29)
- Tweaking Parameters(10:34)
- Aim Walking(8:07)
- Reconciling Aiming and Reloading(10:22)
Section 9: Ammo Pickups
- Ammo Assets(8:07)
- Ammo Class(12:06)
- Overriding SetItemProperties(7:27)
- Pickup Ammo Function(13:01)
- Ammo Widget(12:27)
- Ammo Widget Continued(9:01)
- Bind Ammo Count(3:46)
- Bind Ammo Icon(3:02)
- Pickup Ammo on Overlap(10:58)
- Interpolation Scene Components(17:37)
- Setup Interp Locations(13:39)
- Interp to Multiple Locations(21:59)
- Limit Pickup and Equip Sounds
Section 10: Outline and Glow Effects
- Outline Effect Theory(9:43)
- Post Process Materials(11:43)
- Custom Depth(4:23)
- Texel Position and Size(9:54)
- Show Interior Pixels(13:31)
- Getting the Border(6:09)
- Adding the Border to the Scene Color(6:23)
- Hide Occluded Pixels(7:03)
- Change Outline Thickness(2:02)
- Color Tinting Effect(4:14)
- Multiple Colors with Custom Depth Stencil(15:46)
- Blend Materials with Material Functions(8:48)
- Fresnel Effect on Glow Material(9:33)
- Material Instances(5:10)
- Scrolling Lines Effect(7:41)
- Enable Custom Depth in C++(8:35)
- Dynamic Material Instances(10:05)
- Enable Glow Material in C++(6:20)
- Show Outline While Interping(5:43)
- Curve Vector for Material Parameters(22:06)
- Material Pulse when Interping(16:51)
- Inventory Slot Widget(9:08)
- Inventory Bar Widget(11:17)
- Add Button Icons to the Inventory Bar(9:43)
- Add Inventory Bar to ShooterHUDOverlay(2:24)
- Inventory Array in C++(9:46)
- Binding the Background Icon(13:23)
- Binding the Item Icon(7:53)
- Binding the Ammo Icon(5:31)
- Binding Weapon Ammo Text(4:09)
- Set Item State for Picked Up(7:06)
- Send Slot Index with a Delegate(10:13)
- Play Widget Animation(13:26)
- Exchange Inventory Items(12:45)
- Disable Trace while Interping(14:13)
- Prevent Swapping while Reloading(5:18)
- Equip Montage(12:23)
- Play Equip Sound when Swapping(10:40)
- Swap Pickup Text(7:31)
- Swap Animation Limitations(10:27)
- Create Icon Animation(13:37)
- Create Icon Highlight Delegate(3:14)
- Functions to Broadcast Icon Highlight Delegate(5:03)
- Calling Highlight and UnHighlight Inventory Slot(6:01)
- Assigning the Icon Delegate(19:28)
- Data Tables - FTableRowBase(21:22)
- Accessing Data Table Rows in C++(13:50)
- Setting Widget Colors from Data Table Values(3:41)
- Setting Glow Color from Data Table Value(3:27)
- Set Post Process Highlight Color from Data Table(5:42)
Section 11: Multiple Weapon Types
- Weapon Data Table(13:03)
- Getting Data from Weapon Data Table(19:53)
- Assault Rifle Glow Material(8:24)
- Set Material Instance and Index with Data Table(16:39)
- Adding Barrel Socket to AR(3:42)
- FABRIK IK(11:55)
- Switch on Weapon Type(10:20)
- Disable FABRIK IK when Reloading(7:15)
- AR Reload Animation(6:03)
- Reload Montage Section in Data Table(8:51)
- AR AnimBP(9:56)
- Set AnimBP for Weapon from Data Table(7:07)
- Different Crosshairs Per Weapon(6:57)
- Drawing Crosshairs from Weapon Variables(7:26)
- More Weapon Properties in Data Table(5:02)
- Use Weapon Properties in FireWeapon(8:19)
- Adding Pistol Assets(3:54)
- Hide Bone By Name(7:32)
- Pistol Icon(3:32)
- Pistol Data Table Properties(9:27)
- Barrel Socket on the Pistol(3:09)
- Pistol Reload Animation(9:27)
- Pistol FABRIK IK(4:45)
- Pistol Aiming Pose(8:31)
- Aiming Pose for Each Weapon(7:15)
- Pistol Slide Curve(7:02)
- Pistol Slide Timer(8:38)
- Update Slide Displacement(4:12)
- Transform Pistol Slide Bone(21:37)
- Pistol Glow Material(8:34)
- Semi Automatic Fire(9:32)
- Stop Aiming when Exchanging Weapons(6:02)
Section 12: Footsteps
- Download Footsteps Assets(4:47)
- Setup Assets for Footsteps(17:19)
- Define Physical Surface Types(5:06)
- Creating Physical Materials(9:30)
- Footstep Sync Markers(12:06)
- Custom Anim Notify(10:24)
- Adding Notifies to Animations(22:38)
- Setting Bone Name for Each Notify(8:05)
- Playing Sounds with Anim Notify(3:36)
- Line Trace for Physical Surface Type(12:19)
- The Grass Surface Type(7:25)
- Get Surface Type(8:29)
- Implement Footsteps Notify(12:35)
- Jumping and Landing Sounds(14:06)
- Turing Hips while Running(15:59)
- Turn Hips while Running Backwards(15:34)
Section 13: Multiple Character Meshes
- Retargeting the Animation Blueprint(10:15)
- Setting Up Twin Blast Character Blueprint(10:05)
- Tweaking Twin Blast(10:53)
- Phase(5:26)
Section 14: The Enemy Class
- Enemy Assets(4:12)
- The Enemy Class(3:37)
- Bullet Hit Interface(11:21)
- Implementing BulletHit(17:37)
- Explosive(10:33)
- Damage(14:38)
- Head Shot Damage(7:56)
- Enemy Health Bar(7:55)
- Hide Health Bar(9:07)
- Enemy Death Function(2:18)
- Enemy Anim Instance(3:17)
- EnemyHit Montage(8:38)
- Play Montage Sections(6:28)
- Hit React Delay(6:19)
- Show Hit Numbers(8:56)
- Store Hit Number Locations(4:47)
- Remove Hit Number(9:00)
- Update Hit Number Location(6:12)
- Bind Hit Number Text(3:04)
- Animate Hit Numbers(5:04)
- Head Shots(7:18)
- Grux Physics Asset(3:34)
Section 15: AI and Behavior Trees
- Unreal Engine AI(7:30)
- Adding AI Modules(3:30)
- AI Controller(15:36)
- Creating a Blackboard and Behavior Tree(3:44)
- Patrol Point(7:29)
- Move To Task(14:11)
- Enemy Run Animation
- Second Patrol Point(7:28)
- Wait Task(3:32)
- Agro Sphere(10:01)
- Move To Actor(5:01)
- Stun Enemy
- Stunned Blackboard Decorator(11:10)
- Selector Node(7:30)
- In Attack Range(12:02)
- Enemy Attack Montage(13:16)
- Get Attack Section Name(4:36)
- Custom Behavior Tree Task(13:24)
- Weapon Collision Volumes(13:53)
- Activate and Deactivate Collision(9:47)
- Enemy Damage(11:11)
- Character Health Bar(5:40)
- Enemy Weapon Attack Sounds(3:34)
- Melee Impact Sound(4:56)
- Enemy Vocal Attack Sounds(3:15)
- Weapon Trails(7:29)
- Blood Particles(16:22)
- Character Stun State(8:13)
- End Stun Anim Notify(2:42)
- Stun Character on Hit(8:47)
- Limit Enemy Attacks(9:06)
- Delay Before Chasing the Player(3:11)
- Agro Enemy when Shot(3:19)
- Enemy Death Montage(7:08)
- Destroy Enemy(4:15)
- Polish up Enemy Death(5:55)
- Character Death(10:17)
- Stop Enemy Attack on Death(4:16)
- Retargeting New Montages(3:15)
- New Types of Enemies(8:09)
- Showcasing Different Grux Enemies(9:23)
Section 16: Khaimera
- Adding Khaimera(8:46)
- Khaimera's Animation Blueprint(8:50)
- Khaimera Attack Montage Notifies(8:11)
- Khaimera Death and Hit Montages(5:08)
- Khaimera Anim Notifies(3:14)
- Finishing Khaimera Anim BP(5:41)
- Different Khaimera Skins(5:18)
- Explosive Get Overlapping Actors(12:18)
- Explosive Agro Enemy(11:11)
- Health Pickup(7:43)
Section 17: Level Creation and Finishing the Game!
- Level Prototyping Tools(5:41)
- Level Prototype - Starting Area(7:04)
- Level Prototype - Courtyard(12:45)
- Level Prototype - Courtyard 2(21:05)
- Level Prototype - Using Paragon Props(19:08)
- Ruins Assets - Floor(9:29)
- Ruins Assets - Walls(10:09)
- Ruins Assets - Walls 2(4:22)
- Ruins Assets - Corners(7:54)
- Ruins Assets - Levels(10:14)
- Ruins Assets - Levels 2(8:29)
- Ruins Assets - Levels 3(8:39)
- Ruins Assets - Arches(12:16)
- Ruins Assets - Arches 2(3:27)
- Ruins Assets - Large Stairs(9:05)
- Ruins Assets - Boss Room(20:43)
- Ruins Assets - Finishing Up the Level(6:40)
- Level Design - Enemies(13:14)
- Polishing Gameplay(5:32)
- Polishing Gameplay 2(20:04)
- Prevent Attack when Enemy Dead(1:21)
- Sphere Reflection Capture(3:27)
- Post Process Effects(11:16)
- Esc to Quit(1:50)
- Finishing the Game(10:06)