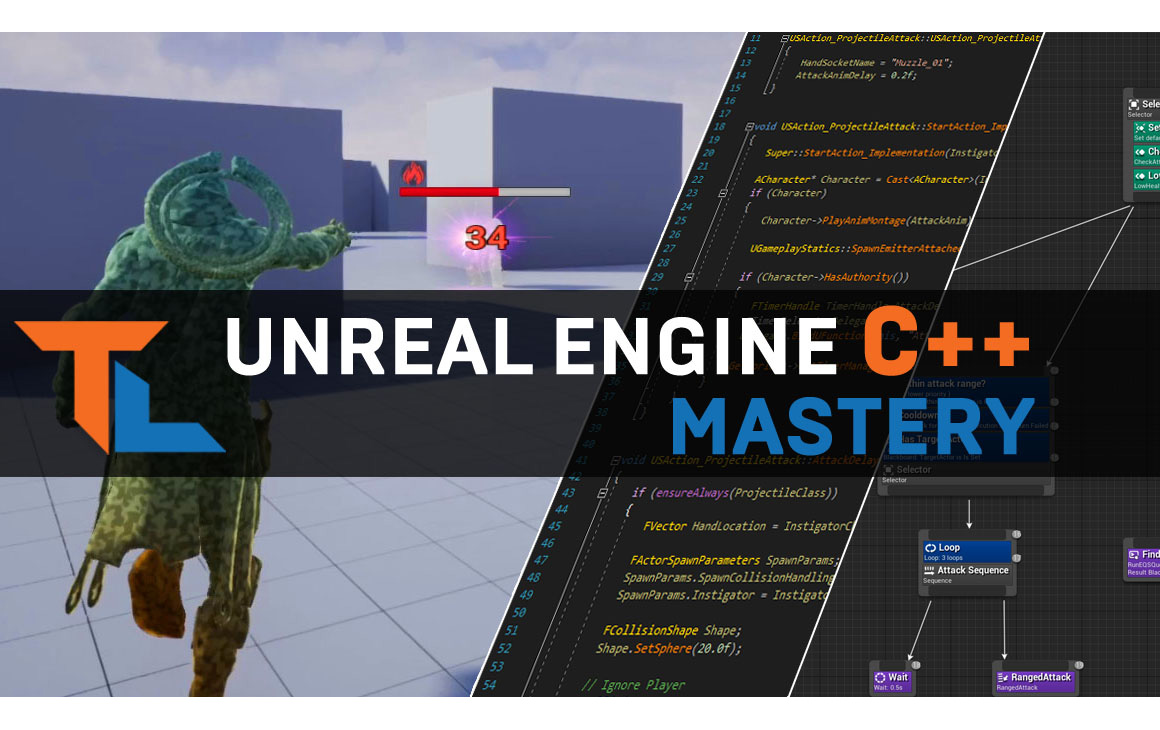
Professional Game Development in C++ and Unreal Engine
The course covers C++ game development for Unreal Engine in detail by building a complete game including an ability system, proper gameplay framework architecture, AI opponents, full multiplayer support and so much more…
Curriculum
- 30 Sections
- 122 Lessons
- 0 Quizzes
- 0m Duration
Lecture 1 - Introduction & Setup
3 Lessons0 Quizzes
- Welcome! (Course Structure)(3:05)
- Source Code Editor Setup Guides (Windows/Mac)
- Finding Information & Answers (Resource Overview)
Lecture 2 - Project Start & Version Control
5 Lessons0 Quizzes
- GitHub Repository and Blank C++ Project(8:54)
- Project Walkthrough and first C++ Class(13:12)
- Adding Components (Third-person Camera)(10:46)
- Binding Movement Input(8:05)
- Character Setup & Course Content (GitHub Commit Example)(4:42)
Lecture 3 - Gameplay, Collision, and Physics
3 Lessons0 Quizzes
- Character Input & Rotations(17:10)
- Magic Projectile Attack(26:10)
- Assignment 1 (Character Setup, Magic Projectile, Explosive Barrel)(1:51)
Lecture 4 - Interfaces & Collision Queries
3 Lessons0 Quizzes
- C++ Interfaces (Interacting with Actors)(12:45)
- ActorComponents & Collision Traces (InteractionComponent)(35:08)
- Animations and Timers (Improving the Attack)(7:49)
Lecture 5 - Blueprints 101
4 Lessons0 Quizzes
- What is Unreal's Blueprint?(5:46)
- Blueprint Interaction & Casting(15:16)
- Animating in Blueprint(14:54)
- Projectile Blueprint & Collision(18:32)
Lecture 6 - Debugging Tools
6 Lessons0 Quizzes
- Assignment 1 Solution Review(10:10)
- "Hotreload" Warning(0:25)
- Debugging C++ & Blueprints (Logging, Breakpoints, Drawing Debug Shapes)(17:26)
- Camera Changes & First UMG Widget (Crosshair)(5:45)
- Assignment 2 (Blackhole Projectile, Dash Ability, Targeting)(10:17)
- 'Asserts' for pro-active detection of bugs(7:19)
Lecture 7 - UMG & (Player) Attributes
5 Lessons0 Quizzes
- Attribute Component (RPG-style)(22:18)
- UMG with Data Bindings (Health Bar)(11:26)
- Event-based principles with UMG (Health Change Events)(16:54)
- Animating UMG Widgets(5:02)
- Dealing Damage with Attributes (Explosive Barrel)(3:03)
Lecture 8 - (Dynamic) Materials for Gameplay
6 Lessons0 Quizzes
- Intro & Projectile Collision Fix(2:50)
- Material Basics ("Shaders")(8:20)
- Hit Flash Material & Driving Material parameters through C++(11:25)
- Simple Dissolve Materials (Textures)(7:28)
- Creating Re-usable "Material Functions"(4:22)
- Materials for User Interface (UMG)(8:34)
Lecture 9 - Audio, Animation, UI
5 Lessons0 Quizzes
- Assignment 2 Solution Review(15:40)
- Creating Sound Cues(7:46)
- Animation Blueprints & Handling Player Death(14:09)
- Projected "3D" UI (Damage Numbers)(10:49)
- Assignment 3 (Health/Damage, Audio, Projectiles, UI)(7:16)
Lecture 10 - Basic AI & Behavior Trees
3 Lessons0 Quizzes
- AI Overview (Behavior Trees, Blackboards, EQS)(5:04)
- Bot Behaviors and Movement(23:24)
- Custom "Check Attack Range" Service for BT(26:14)
Lecture 11 - Intermediate AI with Custom Tasks & EQS
4 Lessons0 Quizzes
- Custom C++ BT Task for Ranged Attacks(17:24)
- Environment Queries for smarter movement(18:53)
- Adding "Sight" with Pawn Sensing Component(12:25)
- Improving Bot Animations & C++ Asserts(7:24)
Lecture 12 - More AI, Environment Query Spawn Logic
4 Lessons0 Quizzes
- Assignment 3 Solution Review(9:37)
- Assignment 4 (Expanding AI Behavior)(1:32)
- EQS to find bot spawnpoints(10:40)
- GameMode with custom AI spawn behavior(29:59)
Lecture 13 - Finalizing AI, Extending the Framework
5 Lessons0 Quizzes
- Further AI improvements (Damage, Death, Ragdolling)(22:46)
- More senses for AI (Reacting to Damage)(6:14)
- Helpful utilities for game framework (static functions)(8:45)
- Improving bot firing logic (weapon accuracy, collision checks)(11:58)
- Damage feedback through Hitflash effect(4:41)
Lecture 14 - UMG With C++ & More Framework Extensions
4 Lessons0 Quizzes
- 3D (Projected) Health bar with C++(29:50)
- Updating HUD Widgets (Health, Credits, GameTime)(15:03)
- Setup Proper Player Spawn(4:42)
- Adding Debug Commands(8:41)
Lecture 15 - Console Variables, GameMode Rules
4 Lessons0 Quizzes
- Assignment 4 Solution Review(4:19)
- Refining Player Respawns
- Console Variables for debugging and game balancing
- Assignment 5 (Credits System, EQS Spawning)(2:22)
Lecture 16 - Writing our own "Gameplay Ability System" alternative
4 Lessons0 Quizzes
- What is "GAS" and why write our own?
- Starting our Action System and first "Action" (Sprinting)(20:24)
- Converting the Projectile Attacks to "Actions"(21:24)
- Improving the Physics Impulses on bot ragdolls(2:42)
Lecture 17 - GameplayTags
4 Lessons0 Quizzes
- Setting up GameplayTags(14:11)
- Comparing Tags in our Action System (Granting and Blocking Tags)(10:47)
- GameplayTags for DOOM-style Door & Keycard system(6:50)
- "Parrying Attacks" using GameplayTags(21:28)
Lecture 18 – Creating "Buffs", World Interaction
4 Lessons0 Quizzes
- Assignment 5 Solution Review(12:03)
- Creating Buffs & Debuffs (Damage over Time Effect)(21:54)
- Improving World Interaction with UI Feedback(15:08)
- Assignment 6 (Rage, Thorns, Power-up, Spotted UI)(3:56)
Lecture 19 – Multiplayer 1 “Network Replication”
3 Lessons0 Quizzes
- What is Network Replication? (Client-Server Model)(15:10)
- Networking the world interaction logic (RPCs)(24:31)
- Networking the Treasure Chest (RepNotify)(13:20)
Lecture 20 – Multiplayer 2
4 Lessons0 Quizzes
- Authority and Actor instances on Clients(8:53)
- Networking the Attributes & UI state (Health)(29:53)
- Blueprint Networking(9:27)
- Networking the Action System(8:55)
Lecture 21 – Multiplayer 3
5 Lessons0 Quizzes
- Assignment 6 Solution Review
- Assignment 7 (Replicating Attributes & Power-ups)(3:13)
- Preparing the Actions with on-screen logging(8:37)
- Networking UObjects & Actions (Action System)(26:31)
- Note on RepNotify behavior(3:32)
Lecture 22 – Finishing up Multiplayer
4 Lessons0 Quizzes
- Code flow and execution between Clients and Server(10:49)
- Limiting Authority of the Client(19:48)
- Networking remaining features (Sprint, etc.)(6:03)
- Improving on Event-based UI(13:50)
Lecture 23 – Serializing Game & Player Progression
5 Lessons0 Quizzes
- Setup core Save/Load Functionality (SaveGame & Slots)(15:32)
- Serializing Player Progression (PlayerState)(11:23)
- Serializing World Transforms (World State #1)(8:49)
- Serializing Any Variables Automatically (World State #2)(14:05)
- Serializing Blueprints & Saving via Actor Interaction(6:42)
Lecture 24 – Building Menus in UMG
4 Lessons0 Quizzes
- Assignment 7 Solution Review(12:05)
- Additional Content .ZIP
- Creating Main Menus with UMG(31:44)
- Creating In-Game Menus(12:13)
Lecture 25 – UMG & Styling Widgets
4 Lessons0 Quizzes
- Setup Widgets & Event-based logic for Buffs/Debuffs(18:18)
- Finishing Buff Bar & Animated materials for UI(15:03)
- Syncing ActionEffect (Duration) for Multiplayer(7:25)
- Widget Styling (HUD & Menus)
Lecture 26 – Animation Blueprints & UI Improvements
3 Lessons0 Quizzes
- Animation State Machines (Stunned Effect) & C++ AnimInstance(21:26)
- Blend Poses & Blend Spaces (Floating Sprint Animation)(14:10)
- Status Text for Interactables (UMG) & C++ Loc Text(17:57)
Lecture 27 – Data Assets, Data Tables, Async Loading (Asset Manager)
3 Lessons0 Quizzes
- Data Tables & Data Assets (Setup for Bot Spawns)(15:26)
- Soft References (Size Map, Reference Viewer)
- Primary Assets & Async Loading (Asset Manager)(19:22)
Lecture 28 – Packaging, Performance, Polish
4 Lessons0 Quizzes
- Cooking Content & Packaging(9:08)
- Profiling Basics & Stat Commands(15:35)
- Unreal Insights for CPU & LoadTimes(12:54)
- Fixing up log warnings and errors(13:02)
Lecture 29 – Wrapping Up
3 Lessons0 Quizzes
- Improving the SaveGame, Collisions & UI(14:55)
- "WARPSQUAD" Project Workflow Demo(19:59)
- Where to go next...(6:52)
Additional Features
4 Lessons0 Quizzes
- Programming with Subsystems (UGameInstanceSubsystem)(5:09)
- Extending Editor & Project Settings (UDeveloperSettings)(4:25)
- Oodle Data Compression (4.27+) (WIP)
- Enhanced Input System (4.27+) (WIP)